태그보기
사진보기
제목보기
태그검색
Search
Implement Optimistic UI for insert/update/delete mutations with React Query
리액트 쿼리로 삽입/갱신/삭제 상태 변경을 위한 Optimistic UI(OUI) 구현
If you wonder what Optimistic UI is, I suggest you check out my previous article Better UX with Optimistic UI.
Optimistic UI가 뭔지 궁금하면 이전 글을 참조해라.
I assume that you’re familiar with React Query. If not, you can check my other article Fetch, Cache, and Update Data Effortlessly with React Query. It is really easy to use. I love React Query! Not only you can control every detail of how you fetch, cache, and use the data you get from the server but also you can implement optimistic updates easily with React Query. You can check out the docs on optimistic updates here.
리액트 쿼리는 잘 알 것이다. 만약 아니라면 이 글을 읽어보라.정말 사용하기 편하다...
모든 세부 사항을 제어할 수 있을 뿐만 아니라 optimistic updates를 쉽게 구현할 수 있다.
Generally, an optimistic update comes with a mutation. A mutation is typically used to insert/update/delete data or perform server side-effects. You’re likely going to have something like this:
일반적으로 OUI은 mutation을 수반한다. mutation은 일반적으로 삽입/갱신/삭제하거나 서버의 side-effects를 수행하기 위해 사용한다.
Like Button
I showed you how to implement a like button in the previous article. Let’s see how we can achieve this with React Query.
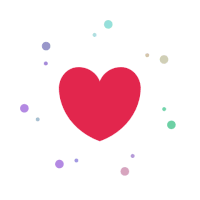
Twitter Like Button
Suppose you have a component that renders the list of tweets using React Query:
Optimistic UI With React Query 정리
2022/03/17
2022/05/05 12:46
2022/03/17 18:18
1.
classnames를 사용하지 않을 때
2.
classnames 사용
3.
classnames/bind 사용
Example 3: with classnames.bind
In the above example, we're using the styles object every time we need to reference a local classname. This can be quite cumbersome. If you're converting an old component to use CSS modules you can actually increase the files size (and reduce the readability) quite a bit.
Thankfully, classnames ships with an alternative bind method that's designed specifically to make working with CSS modules much less cumbersome. It requires a small bit of extra set up (and has one important caveat) but it gets us closer to the less verbose syntax we enjoyed in the days before CSS Modules. Of course, there are numerous benefits of using CSS Modules. So, why not make it easy to work with?
위의 예에서는 로컬 클래스 이름을 참조해야 할 때마다 styles 객체를 사용한다. 이것은 꽤 번거로울 수 있다. 오래된 구성요소를 CSS 모듈을 사용하도록 변환하는 경우 실제로 파일 크기를 크게 늘리거나 가독성을 낮출 수 있다.
다행히 클래스 네임은 CSS 모듈 작업을 훨씬 덜 번거롭게 하기 위해 특별히 설계된 대체 바인드 방법을 제공한다. 약간의 추가 설정이 필요하지만(중요한 주의사항이 하나 있음) CSS Modules 이전 시대에 즐겼던 덜 장황한 구문에 더 가까워진다.
Bad things:
[classnames] classnames/bind 함수와 classnames 함수의 차이
2022/03/13 16:00
2022/03/13 14:48
What does classnames/bind get us over treating the imported styles like normal classes (which they are)?
클래스 이름/바인드가 가져온 스타일을 일반 클래스처럼 취급하면 도움이 되는 것은 무엇입니까?
Could write
저는 https://github.com/gajus/babel-plugin-react-css-modules.에 대해 잘 모릅니다. readme를 잠깐 훑어보니 styleName 특성이 추가된 것 같습니까? className과 같은 표준 React API를 사용하는 것이 낫습니다.
Less magic = More better.
Also, I'd encourage the use of camel case class names. For example
Slightly less typing.
The main reason for not using camel case was to implement some form of BEM so we write our classes like this block__element--modifier but since the files should remain mostly minimal, I'm open to avoiding that. The other factor is that classnames handles the concatenation for us and allows us to do checks without dealing with ternaries etc.
For the styleName piece, I'm pretty solidly against it at this point. Having used both now, I can confidently say the added syntactic sugar isn't worth the real added complexity.
classnames.bind로 css modules를 전부 대체해서 스타일 1개일 때도 적어야 할까?
2022/03/13 16:00
2022/03/13 14:48
당신과 나의 미래가 React 앱에서 캔버스 요소를 설정하는 시간을 절약할 수 있도록, HTML 캔버스를 React 후크와 함께 사용하는 방법에 대한 최종 버전을 공유하겠습니다.
1단계: 캔버스 요소 렌더링
width및 height속성은 캔버스 요소에 의해 생성된 이미지에 대해
이미지 해상도와 종횡비(image resolution and the aspect ratio)라는 두 가지를 결정합니다 .
이미지 해상도 Image resolution
이미지에는 100 x 100 픽셀이 있습니다. 이 경우 이미지 너비의 1/100보다 얇은 선을 그리면 하위 픽셀 렌더링으로 끝나므로 성능상의 이유로 인해 이를 피해야 합니다(MDN 기여자 2019b 참조). 가장 얇은 선이 예를 들어 이미지 너비의 1/200이면 너비="200"을 설정해야 합니다.
종횡비 Aspect ratio
위의 예에서는 영상의 가로 세로 비율을 1:1(즉, 정사각형)로 정의합니다.
너비 및 높이 속성을 지정하지 못한 경우(HTML 캔버스의 많은 문서처럼), 기본 가로 세로 비율은 2:1(너비 300px 및 높이 150px)이 적용됩니다.
Corey의 (2019) - React 후크를 사용하여 캔버스 요소를 렌더링하는 방법에 대한 유용한 기사는 width및 height속성을 지정하지 않아 이 함정에 빠진 것으로 보입니다.
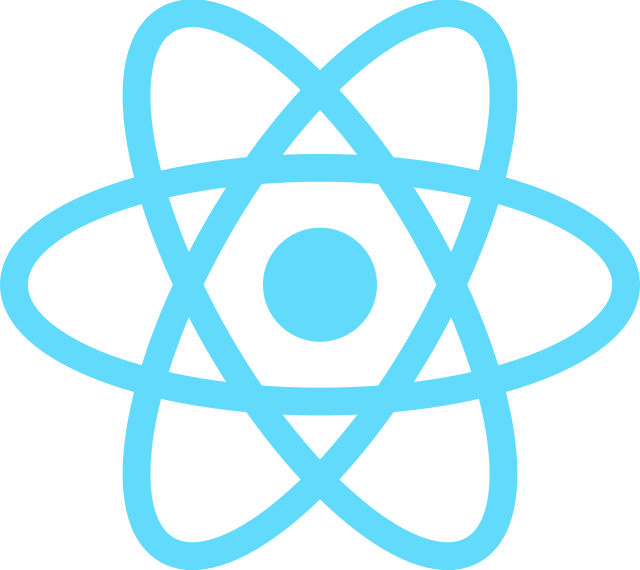
리액트와 캔버스 호환
2022/03/13 15:53
2022/03/13 14:49
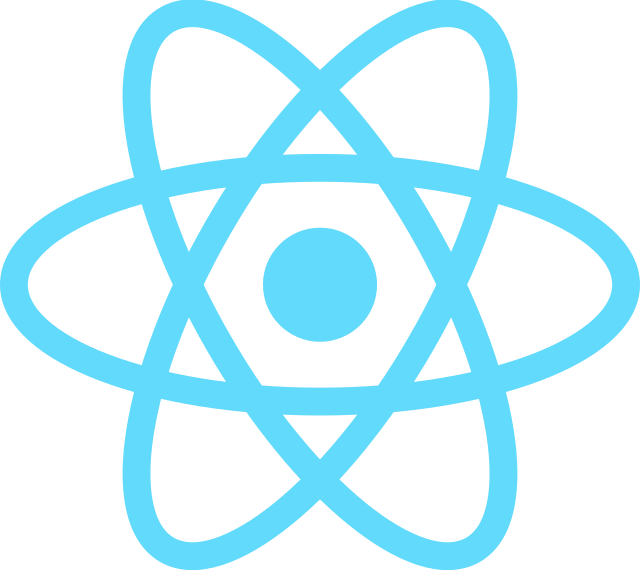
리액트에서 useRef를 통해 참조되는 HTML canvas element의 매개변수를 저장하는 가장 좋은 방법은 무엇인가
2022/03/13 15:53
2022/03/13 14:49
Recently, How to Create the Drawing Interaction on DEV's Offline Page by Ali Spittel showed up in my feed and it looked quite cool. This got me wondering if I could create the same thing as a React component using Hooks and typescript. Well, the fact that I am writing this post means I was able to recreate it. So let's see how I did it.
드로잉 인터랙션을 만드는 방법이 등장했는데 꽤 멋져 보였다. 이것은 내가 Hooks와 typescript를 사용하여 React 컴포넌트와 같은 것을 만들 수 있는지 궁금하게 만들었다.
If you are interested in the final product, you can check out the Github repository. There is also a sandbox you can play with at the end of this post.
This post assumes you already know how to work with TypeScript and hooks.
Creating the Component
The first thing we need to do is to create a Canvas component. The canvas needs to take up some space which we will want any parent component to be able to override so we will add width and height as props.
But, We want to add a sensible default so that We don't have to add these props every time we want to use this component. We will add some defaultProps to set these values to window.innerWidth and window.innerHeight respectively.
우리가 가장 먼저 해야 할 일은 캔버스 컴포넌트를 만드는 것이다. 캔버스가 공간을 차지해야 하는데, 상위 컴포넌트가 "오버라이드" 가능하기 때문에 width과 height를 props로 추가할 것이다.
하지만 우리는 이 컴포넌트를 사용할 때마다 이러한 props를 추가할 필요가 없도록 sensible default를 추가하고 싶습니다.
이러한 값을 각각 window.innerWidth 및 window.innerHeight로 설정하는 defaultProps를 추가합니다.
Lets Draw
Since we need to modify the canvas element, we will need to add a ref to it. We can do this by using useRef hook and modifying our canvas element to set the ref.
캔버스 요소를 수정해야 하므로 ref를 추가해야 합니다. useRef를 사용하여 ref를 설정한 캔버스 요소를 수정한다.
Set state
We need to keep track of some variables
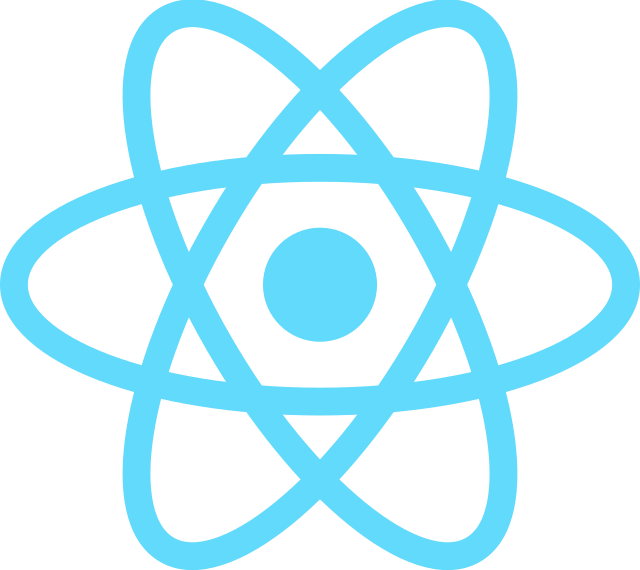
React Component to Draw using Hooks and Typescript(2020)
2022/03/13 15:53
2022/03/13 14:49
There is no “right” answer for this. Some users prefer to keep every single piece of data in Redux, to maintain a fully serializable and controlled version of their application at all times. Others prefer to keep non-critical or UI state, such as “is this dropdown currently open”, inside a component's internal state.
Using local component state is fine. As a developer, it is your job to determine what kinds of state make up your application, and where each piece of state should live. Find a balance that works for you, and go with it.
Some common rules of thumb for determining what kind of data should be put into Redux:
•
Do other parts of the application care about this data?
•
Do you need to be able to create further derived data based on this original data?
•
Is the same data being used to drive multiple components?
•
Is there value to you in being able to restore this state to a given point in time (ie, time travel debugging)?
•
Do you want to cache the data (ie, use what's in state if it's already there instead of re-requesting it)?
I've talked to people who chose to put literally everything into Redux. You can do that, and I can understand why you might want to do that, but I personally think that's way too dogmatic.
Part of the issue is that Redux's principle of "a single source of truth" gets over-interpreted to mean that you must put everything in Redux, which isn't the case. It's really more like "a single source of truth, for the data that you decide is worth keeping in Redux".
I think much of the confusion around using Redux is because people don't understand some of the background behind it. I wrote a two-part post, The Tao of Redux, Part 1 - Implementation and Intent and The Tao of Redux, Part 2 - Practice and Philosophy, which tries to explain that background and add context. These two posts look at the history and intent behind Redux's design, how it's meant to be used, why common usage patterns exist, and some of the other ways that you can use Redux.
If anyone's interested, my React/Redux links list has sections on React State Management and Redux Architecture, which point to a lot of additional info on this kind of question.
Global state VS local state 리덕스의 배경이해, 글로벌 변수와 다를 바 없다면 지양되어야 하지 않나?
2022/03/13 15:58
2022/03/13 14:51
1. Testing and the frontend
Test-driven development (‘TDD’) is in the mouths of every backend developer, as testing has finally assumed its rightful position as a ‘must-have’ of software engineering. Any company not utilising backend testing is behind the curve. However, this surge in interest has only recently begun picking up steam for the frontend. Anyone transitioning to frontend development is likely to feel very comfortable with unit testing, where specific components are checked to ensure they’re producing the right outputs. However, frontend E2E testing is an entirely different beast.
1. 테스트 및 프런트엔드
The key concept to understand in frontend testing is that we want to test results, not implementation details. In other words: does the screen end up looking how we want it to look, and doing what we want it to do? Great! We don’t care about specific function calls, state, or data structures here. We’re simply testing the user experience.
2. Arrange/Act/Assert
Arrange/Act/Assert is a simple way to approach testing, which can help you understand the basic process by which all tests (including backend!) are structured. They also form a good basis for explaining the basics as we move through our demo application. In short, Arrange/Act/Assert is a framework for structuring your tests.
The first step when writing a test is to ‘arrange’ the right environment. In our case, you’ll see we need to load up the page we want to test.
2. Arrange/Act/Assert
Next, we ‘act’ by doing an action (or series of actions) necessary to replicate how the user could interact with our app. Another way of thinking about it is that the ‘Act’ is the action we’re testing.
But of course, there’s no point loading a page, entering some data, and clicking “Submit” without actually telling our tests what we expect. Do we want Submit to result in a popup or a page load? This is where ‘assert’ comes in: we tell our test file exactly what outcome we expect, based on the actions in the previous steps.
3. Introducing our example app
For the sake of this demo, I’ve created a small ‘MadLibs’ app with React. For those unfamiliar with MadLibs as a concept, it involves writing a short story with some gaps in it, filled with words entered by the user. The catch? The user doesn’t know how the words will be used! They enter the words according to what is needed (‘animal’, ‘food’, etc.) and then the author assembles them in a way which can produce some really weird and wonderful stories.
• Example MadLibs app without Cypress: https://github.com/AJMcDee/Cypress_Demo_BaseApp
Implementing Your First End-to-End Tests In React Using Cypress
2022/03/13 16:00
2022/03/13 14:52
Load more